
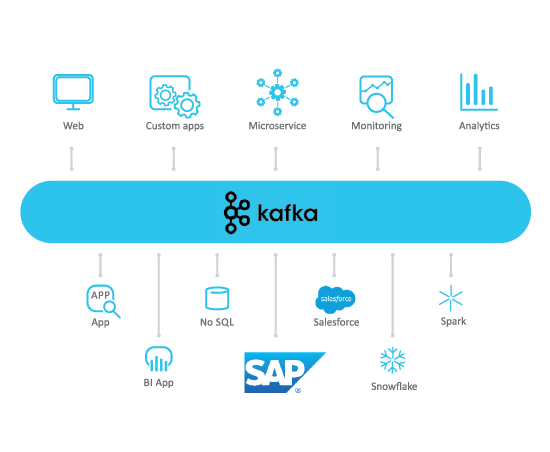
Note that our value serializer will automatically convert and encode the data. This can be done by calling the send method on the producer and specifying the topic and the data. Within the same loop we will also send our data to a broker. This is not the topic key, but just a key of our data. This can be done with a for-loop where we feed each number as the value into a dictionary with one key: number.

Now, we want to generate numbers from one till 1000. Producer = KafkaProducer(bootstrap_servers=, value_serializer=lambda x: dumps(x).encode('utf-8')) Here, we convert the data to a json file and encode it to utf-8. value_serializer=lambda x: dumps(x).encode(‘utf-8’): function of how the data should be serialized before sending to the broker.It is not necessary to set this here, since the default is localhost:9092. bootstrap_servers=: sets the host and port the producer should contact to bootstrap initial cluster metadata.from time import sleep from json import dumps from kafka import KafkaProducer This is a great way to make sure all the data is fed into the database without duplicates or missing data.Ĭreate a new Python script named producer.py and start with importing json, time.sleep and KafkaProducer from our brand new Kafka-Python library. The advantage of using Kafka is that, if our consumer breaks down, the new or fixed consumer will pick up reading where the previous one stopped. Then a consumer will read the data from the broker and store them in a MongoDb collection. In our example we’ll create a producer that emits numbers from 1 to 1000 and send them to our Kafka broker. This can be important if you want to consume a topic in parallel with different consumers. a group of consumers subscribed to the same topic, they can commit their offset. If the consumer is part of a consumer group, i.e. In our example we can make abstraction of the partitions, since we’re working locally.Ĭonsumers read the messages of a set of partitions of a topic of their choice at their own pace. Producers always write new messages at the end of the log. Topics are logs that receive data from the producers and store them across their partitions. It is possible to attach a key to each message, in which case the producer guarantees that all messages with the same key will arrive to the same partition. Producers produce messages to a topic of their choice. In the simplest way there are three players in the Kafka ecosystem: producers, topics (run by brokers) and consumers. Simply put, Kafka is a distributed publish-subscribe messaging system that maintains feeds of messages in partitioned and replicated topics. We are also using a topic called numtest in this example, you can create a new topic by opening a new command prompt, navigating to …/kafka/bin/windows and execute: kafka-topics.bat -create -zookeeper localhost:2181 -replication-factor 1 -partitions 1 -topic numtest What is Kafka? In this example we assume that Zookeeper is running default on localhost:2181 and Kafka on localhost:9092.
#Kafka data generator install
pip install kafka-python conda install -c conda-forge kafka-pythonĭon’t forget to start your Zookeeper server and Kafka broker before executing the example code below. You can do this using pip or conda, if you’re using an Anaconda distribution.
#Kafka data generator windows
For Windows there is an excellent guide by Shahrukh Aslam, and they definitely exist for other OS’s as well. First of all you want to have installed Kafka and Zookeeper on your machine.
